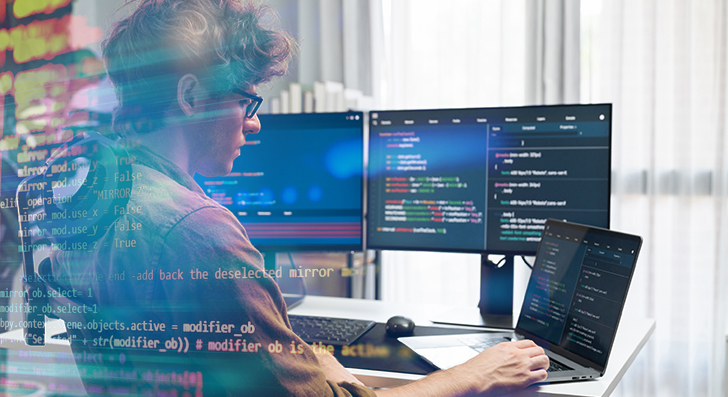
Scalability suggests your software can tackle advancement—far more users, additional knowledge, and a lot more site visitors—without breaking. To be a developer, constructing with scalability in mind will save time and strain later on. Here’s a transparent and sensible guideline that can assist you begin by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability is not a little something you bolt on later on—it ought to be element of your prepare from the beginning. A lot of applications fall short after they mature quickly for the reason that the initial structure can’t manage the additional load. As being a developer, you'll want to Feel early regarding how your method will behave stressed.
Get started by developing your architecture being flexible. Keep away from monolithic codebases where by every little thing is tightly connected. As an alternative, use modular style and design or microservices. These patterns break your application into lesser, independent elements. Just about every module or service can scale By itself without the need of affecting The entire technique.
Also, give thought to your databases from day a single. Will it need to have to handle 1,000,000 end users or merely 100? Choose the right sort—relational or NoSQL—determined by how your facts will mature. Program for sharding, indexing, and backups early, Even though you don’t will need them nonetheless.
Another essential stage is in order to avoid hardcoding assumptions. Don’t publish code that only performs underneath present circumstances. Take into consideration what would take place When your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use design styles that guidance scaling, like message queues or celebration-pushed programs. These enable your application take care of far more requests devoid of obtaining overloaded.
Whenever you Construct with scalability in mind, you're not just preparing for fulfillment—you might be lessening future problems. A nicely-planned method is easier to take care of, adapt, and grow. It’s far better to prepare early than to rebuild afterwards.
Use the ideal Databases
Selecting the ideal databases can be a crucial A part of building scalable purposes. Not all databases are designed precisely the same, and using the Completely wrong one can gradual you down as well as result in failures as your application grows.
Start out by knowledge your information. Is it remarkably structured, like rows in the table? If Indeed, a relational database like PostgreSQL or MySQL is a great match. These are solid with associations, transactions, and regularity. They also aid scaling tactics like read replicas, indexing, and partitioning to handle far more visitors and facts.
Should your details is much more adaptable—like user action logs, product catalogs, or paperwork—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing huge volumes of unstructured or semi-structured details and may scale horizontally additional effortlessly.
Also, look at your study and publish patterns. Will you be performing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases that can cope with high compose throughput, or maybe party-primarily based info storage devices like Apache Kafka (for non permanent data streams).
It’s also wise to Consider in advance. You may not want State-of-the-art scaling options now, but choosing a database that supports them indicates you won’t want to change later on.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your info according to your entry designs. And constantly watch databases effectiveness while you improve.
Briefly, the proper database depends on your app’s composition, velocity requires, And exactly how you be expecting it to improve. Acquire time to choose correctly—it’ll preserve many issues later on.
Enhance Code and Queries
Quick code is key to scalability. As your application grows, just about every smaller delay adds up. Poorly penned code or unoptimized queries can decelerate general performance and overload your procedure. That’s why it’s essential to Create effective logic from the start.
Get started by producing clear, easy code. Avoid repeating logic and take away just about anything unwanted. Don’t select the most complex Alternative if an easy 1 works. Maintain your functions shorter, centered, and easy to check. Use profiling instruments to discover bottlenecks—places wherever your code will take too very long to run or takes advantage of excessive memory.
Next, check out your database queries. These generally sluggish things down in excess of the code itself. Ensure that Every question only asks for the data you really need. Keep away from SELECT *, which fetches almost everything, and instead decide on certain fields. Use indexes to hurry up lookups. And steer clear of executing too many joins, Specifically throughout big tables.
In case you notice the identical details becoming requested repeatedly, use caching. Keep the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your database operations once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and would make your application more effective.
Remember to examination with massive datasets. Code and queries that do the job fine with 100 information may well crash whenever they have to manage 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques enable your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it has to handle much more customers and even more site visitors. If almost everything goes by way of one particular server, it is going to speedily become a bottleneck. That’s in which load balancing and caching are available in. These two equipment aid keep your app speedy, secure, and scalable.
Load balancing spreads incoming website traffic throughout several servers. As opposed to a single server performing all the work, the load balancer routes buyers to unique servers dependant on availability. This suggests no solitary server gets overloaded. If one server goes down, the load balancer can mail traffic to the Other people. Tools like Nginx, HAProxy, or cloud-centered remedies from AWS and Google Cloud make this simple to set up.
Caching is about storing facts briefly so it can be reused immediately. When buyers request exactly the same information and facts yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it within the cache.
There are 2 common types of caching:
one. Server-side caching (like Redis or Memcached) merchants information in memory for rapid accessibility.
two. Client-aspect caching (like browser caching or CDN caching) shops static documents close to the consumer.
Caching reduces database load, increases velocity, and helps make your application much more successful.
Use caching for things which don’t alter generally. And usually ensure that your cache is updated when knowledge does change.
In a nutshell, load balancing and caching here are very simple but effective instruments. Together, they assist your app manage additional customers, remain speedy, and recover from troubles. If you propose to grow, you will need both equally.
Use Cloud and Container Tools
To construct scalable apps, you would like tools that allow your application grow effortlessly. That’s the place cloud platforms and containers can be found in. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure let you rent servers and providers as you may need them. You don’t really need to buy hardware or guess long term capability. When site visitors will increase, you may insert additional means with just some clicks or quickly applying vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and security tools. You are able to concentrate on building your application in lieu of running infrastructure.
Containers are A different essential Device. A container packages your app and all the things it ought to operate—code, libraries, settings—into one device. This causes it to be straightforward to move your app concerning environments, from a laptop computer towards the cloud, without surprises. Docker is the preferred Device for this.
When your application works by using several containers, tools like Kubernetes assist you take care of them. Kubernetes handles deployment, scaling, and recovery. If one aspect of the app crashes, it restarts it automatically.
Containers also help it become simple to different portions of your app into expert services. You'll be able to update or scale parts independently, and that is great for general performance and dependability.
To put it briefly, employing cloud and container tools suggests you are able to scale rapid, deploy very easily, and Get better promptly when difficulties materialize. If you'd like your application to develop devoid of limits, start off using these equipment early. They help you save time, minimize hazard, and assist you to keep centered on developing, not repairing.
Observe Every little thing
When you don’t monitor your software, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location problems early, and make greater conclusions as your application grows. It’s a important Portion of making scalable units.
Begin by tracking standard metrics like CPU use, memory, disk House, and reaction time. These tell you how your servers and companies are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application far too. Regulate how much time it takes for users to load pages, how often errors occur, and exactly where they take place. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for critical challenges. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you need to get notified instantly. This assists you fix issues speedy, generally in advance of end users even recognize.
Monitoring is also practical any time you make alterations. Should you deploy a brand new feature and see a spike in faults or slowdowns, you may roll it back again before it results in true injury.
As your application grows, website traffic and info improve. Without the need of monitoring, you’ll miss indications of problems until it’s far too late. But with the correct tools set up, you stay on top of things.
In brief, checking aids you keep the app reliable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it works well, even under pressure.
Remaining Ideas
Scalability isn’t only for large providers. Even tiny applications require a robust foundation. By planning carefully, optimizing properly, and utilizing the correct instruments, you are able to Create applications that expand efficiently with out breaking under pressure. Get started modest, think huge, and Make intelligent.